MagicDraw API Code: Creating blocks with a simple directed composition relationship.
In this example using MagicDraw API code, we will create one block that represents a parent and another that represents the child or part.
The MagicDraw API code provides the ability to stereotype our created element as “Block” to inherit the appropriate attributes.
Next we create an Association relationship that we set as a Composite with the parent. Because we want a compositional relationship, we apply the appropriate “Part Property” and “Reference Property” stereotypes to the property ends. Also, we set the “Navigable End” value for each to “TRUE” as well as the “Visibility” to “PUBLIC”.
Because our public function call is creating our block elements under a package called “Structure”; you will notice that the Compositional relationship is also owned by this package.
And finally you can use this class with any java script you write to create a compositional hierarchy! Leveraging these basic functions here should provide anyone with the foundation to begin automating in a MagicDraw model.
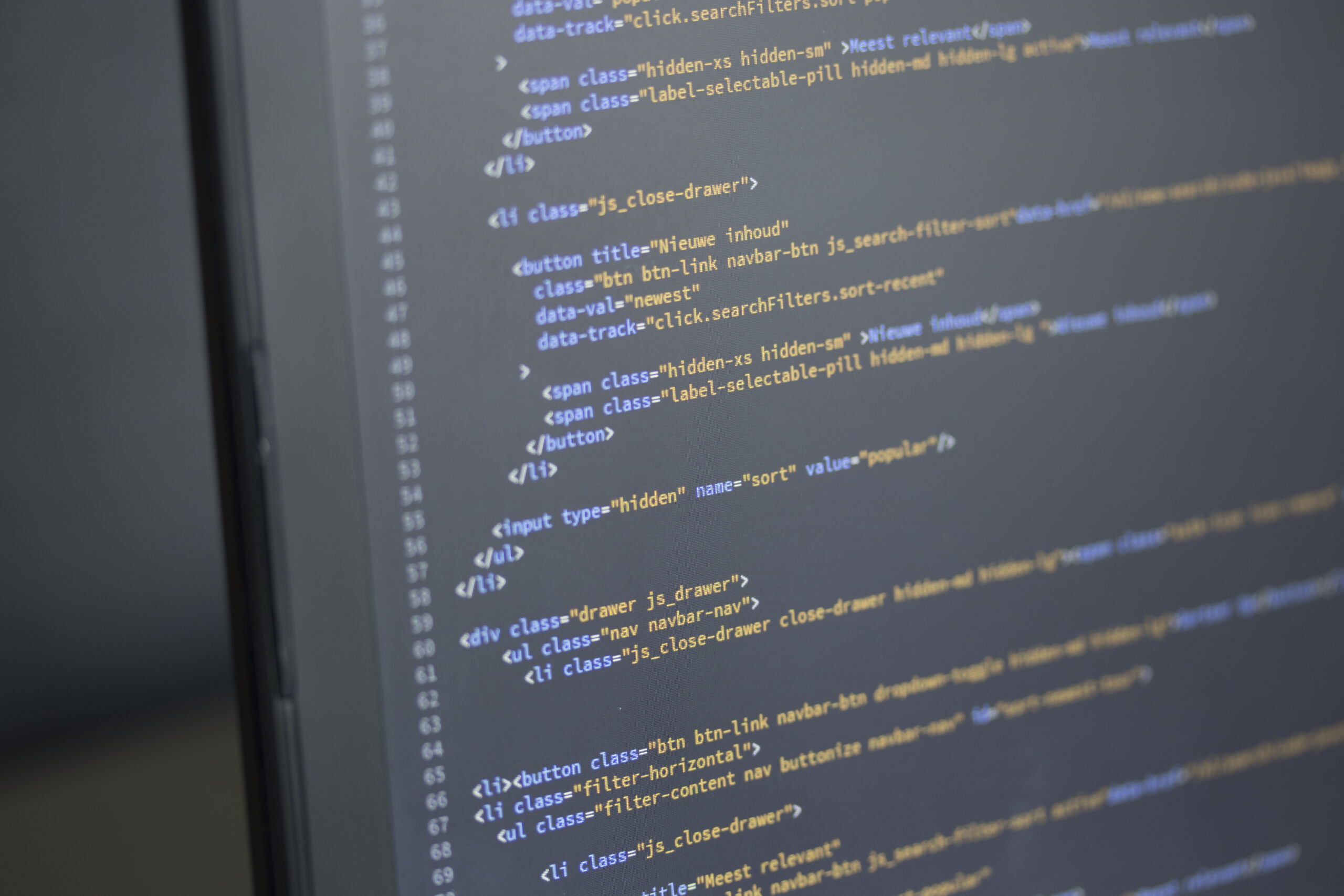
Useful Links
Related Videos
//Create Blocks with Composition Java Class
//Author: Beyond MBSE
//Created for You!
//Insert your package name here
package beyondmbse;
//Import these packages
import com.nomagic.magicdraw.core.Project;
import com.nomagic.magicdraw.uml.Finder;
import com.nomagic.uml2.ext.jmi.helpers.StereotypesHelper;
import com.nomagic.uml2.ext.jmi.helpers.ModelHelper;
import com.nomagic.uml2.ext.magicdraw.classes.mdkernel.Association;
import com.nomagic.uml2.ext.magicdraw.classes.mdkernel.Property;
import com.nomagic.uml2.impl.ElementsFactory;
public class CreateBlockCompositionTool {
private Project my_magicdraw_project = Application.getInstance().getProject();
private Package structure_package = element_factory_instance.createPackageInstance();
public CreateBlockCompositionTool(String parent_name, String child_name)
{
structure_package.setOwner(my_project.getPrimaryModel());
structure_package.setName("Structure");
NamedElement parent_block = createBlock(parent_name, structure_package);
NamedElement child_block = createBlock(child_name, structure_package);
compositionalRelationship(structure_package, parent_block, child_block);
}
private NamedElement createBlock(String name_of_block, Package block_package)
{
NamedElement block = element_factory_instance.createClassInstance();
StereotypesHelper.addStereotypeByString(block, "Block");
block.setOwner(block_package);
block.setName(name_of_block);
return block;
}
private void compositionalRelationship(Package composition_package, NamedElement parent, NamedElement child)
{
Association composition = element_factory_instance.createAssociationInstance;
composition.setOwner(composition_package);
Property parent_end = ModelHelper.getFirstMemberEnd(composition);
StereotypesHelper.addStereotypeByString(parent_end, "PartProperty");
parent_end.setOwner(parent);
parent_end.setName(child.getName());
parent_end.setType((Type) child);
parent_end.setVisibility(VisibilityKindEnum.PUBLIC);
parent_end.setAggregation(AggregationKindEnum.COMPOSITE);
ModelHelper.setNavigable(parent_end, true);
Property child_end = ModelHelper.getSecondMemberEnd(composition);
StereotypesHelper.addStereotypeByString(child_end, "ReferenceProperty");
child_end.setOwner(child);
child_end.setName(parent.getName()); //be sure to create a function that transforms the first part
child_end.setType((Type) parent); //of the parent name string to lowercase
child_end.setVisibility(VisibilityKindEnum.PUBLIC);
ModelHelper.setNavigable(child_end, true);
}
Pingback: Block - Beyond MBSE