MagicDraw API Code Activity: Creating Activity with Call Behavior Actions and Control Flows
In this example we will create an activity element that contains call behavior actions and control flows.
The following classes in the MagicDraw API Code Activity are required:
Because a project may be behavior driven, an automated method for creating activities can help. The code looks daunting at first, so once it’s executed…it will make sense!
The below class begins by creating a package named “Behavior”. All elements created are owned by this package. The createActivity
function takes the following String inputs:
- Activity name
- Initial Node name
- Final Flow Node name
- Call Behavior Action names
The activity
variable is the activity element that will own the call behavior actions, initial node, final flow node and control flows.
A counter
variable is used to determine the beginning and end of the String List of action names. The activity_start
variable is used for the Initial Node. Also, the activity_end
variable is used for the Final Flow Node.
The class contains private functions to aid in the creation of the above variables. These private functions can be changed to public if there is a need to do so. When the createActivity
function completes, it returns the activity
variable.
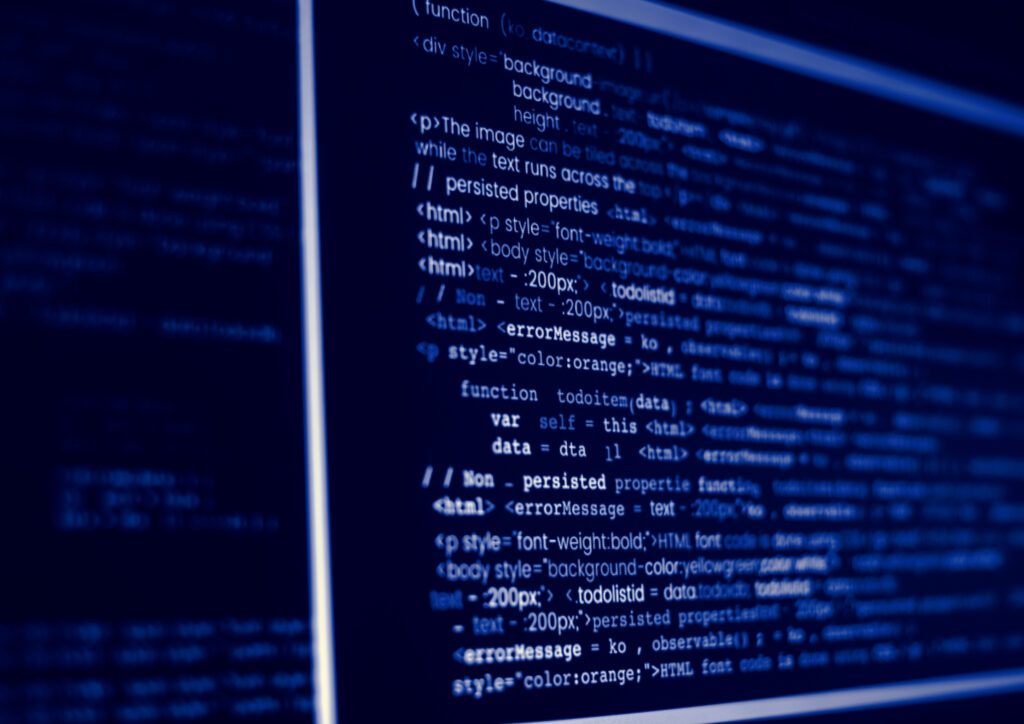
Useful Links
Related Videos
//Create an Activity with Call Behavior Actions and Control Flows
//Author: Beyond MBSE
//Created for You!
//Insert your package name here
package beyondmbse;
//Import these packages
import com.nomagic.magicdraw.core.Application;
import com.nomagic.magicdraw.core.Project;
import com.nomagic.magicdraw.uml.Finder;
import com.nomagic.uml2.ext.magicdraw.activities.mdfundametalactivities.ActivityNode;
import com.nomagic.uml2.ext.magicdraw.activities.mdbasicactivities.InitialNode;
import com.nomagic.uml2.ext.magicdraw.activities.mdintermediateactivities.FlowFinalNode;
import com.nomagic.uml2.ext.magicdraw.activities.mdbasicactivities.ControlFlow;
import com.nomagic.uml2.impl.ElementsFactory;
public class CreateActivityControlFlowTool
{
private Project my_magicdraw_project = Application.getInstance().getProject();
private ElementsFactory element_factory_instance = my_magicdraw_project.getElementsFactory();
private Package behavior_package = element_factory_instance.createPackageInstance();
public CreateActivityControlFlowTool()
{
//instantiate class
behavior_package.setOwner(my_project.getPrimaryModel());
behavior_package.setName("Behavior");
}
public NamedElement createActivity(String activity_name, String initial_node_name, List<String> action_steps, String final_node_name)
{
NamedElement activity = createActivity(String activity_name);
InitialNode activity_start = createInitialNode(initial_node_name, activity);
FlowFinalNode activity_end = createFinalNode(final_node_name, activity);
int counter = 0;
while (counter < action_steps.size()) {
NamedElement action = createCallBehaviorAction(action_steps.get(counter), activity);
if (counter == 0) {
createControlFlow((NamedElement) activity_start, action, activity);
} else {
NamedElement previous_action = Finder.byNameRecursively().find(behavior_package, element_factory_instance.createCallBehaviorActionInstance().getClassType(),
action_steps.get(counter - 1));
createControlFlow(previous_action, action, activity);
}
int action_steps_end = counter + 1;
if (action_steps_end >= action_steps.size()) {
createControlFlow(action, (NamedElement) activity_end, activity);
} else {
//not the end of the action steps list
}
counter++;
}
return activity;
}
public NamedElement createActivity(String activity_name)
{
NamedElement activity = element_factory_instance.createActivityInstance();
activity.setOwner(behavior_package);
activity.setName(activity_name);
return activity;
}
private InitialNode createInitialNode(String initial_node_name, NamedElement owning_activity)
{
InitialNode flow_start = element_factory_instance.createInitialNodeInstance();
flow_start.setOwner(owning_activity);
flow_start.setName(initial_node_name);
return flow_start;
}
private FlowFinalNode createInitialNode(String final_node_name, NamedElement owning_activity)
{
FlowFinalNode flow_end = element_factory_instance.createFlowFinalNodeInstance();
flow_end.setOwner(owning_activity);
flow_end.setName(final_node_name);
return flow_end;
}
private NamedElement createCallBehaviorAction(String action_name, NamedElement owning_activity)
{
NamedElement action = element_factory_instance.createCallBehaviorActionInstance();
action.setOwner(owning_activity);
action.setName(action_name);
return action;
}
private ControlFlow createControlFlow(NamedElement first_end, NamedElement second_end, NamedElement owning_activity)
{
ControlFlow control_flow = element_factory_instance.createControlFlowInstance();
control_flow.setOwner(owning_activity);
control_flow.setSource((ActivityNode) first_end);
control_flow.setTarget((ActivityNode) second_end);
LiteralUnlimitedNatural designation = element_factory_instance.createLiteralUnlimitedNaturalInstance();
designation.setValue(1);
designation.setOwner(control_flow);
designation.setVisibility(VisibilityKindEnum.PUBLIC);
return control_flow;
}
}
Pingback: Activity - Beyond MBSE
Having trouble to create Directed Association in 2021x Version.
Can someone help